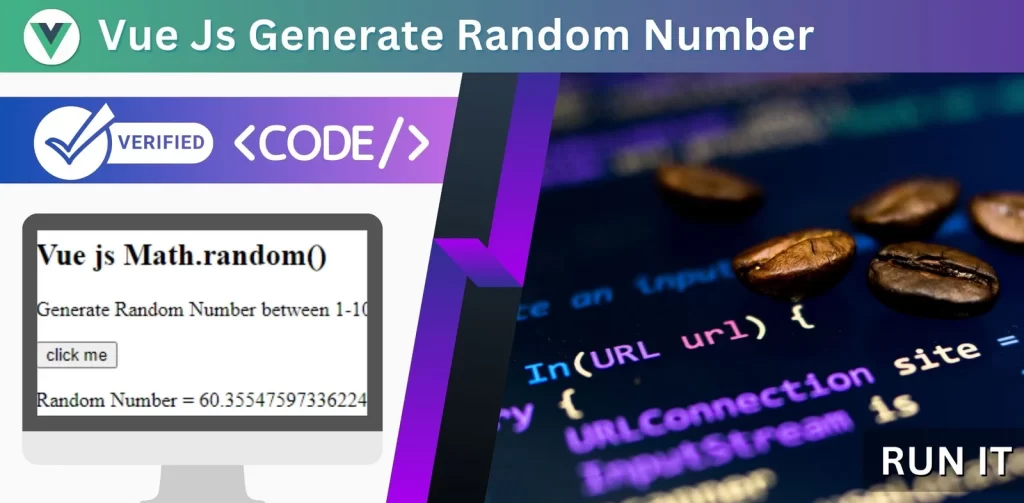
Vue Js Generate Random Number: Vue.js has a Math.random() function that can be used to generate a random number in JavaScript code. Vue Js’s math.random() function is a convenient way to generate random numbers within a given range.This means that the returned random number is always greater than or equal to 0, but it is always less than 1. In this tutorial will teach you how to generate a random number from 1 to 100 using native JavaScript and Vue.js, as shown below.
Vue Js Generate Random Number Syntax
this.randomNumber = Math.random()*100;
How can you generate random numbers in Vue.js ?
Generating random numbers between 1 and 100 in vue.js is relatively simple and can be achieved using the Math.random() method.
Vue Js Generate Random Number between 1 – 100 | Example
<div id="app">
<button @click="myFunction">click me</button>
<p>Random Number = {{randomNumber}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
randomNumber:'',
}
},
methods:{
myFunction(){
this.randomNumber = Math.random()*100;
},
}
}).mount('#app')
</script>
Output of above example

How to generate random string in Vue Js?
Generating random strings can be useful in a variety of situations, such as when creating an alphanumeric password or when generating a unique identifier. In this example, we will learn how to generate random strings by using vue js and native javascript.
Vue Js Generate Random String | Example
<div id="app">
<input type="number" v-model="strLength">
<button @click="myFunction">click me</button>
<p>Random String = {{randomString}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
alphabet:'abcdefghijklmnopqrstuvwxyz',
strLength:"",
randomString:'',
}
},
methods:{
myFunction(){
this.randomString = ' ';
for(var i = 0; i <this.strLength;i++){
this.randomString += this.alphabet.charAt(Math.floor(Math.random()*this.alphabet.length))
}
},
}
}).mount('#app')
</script>
Output of above example
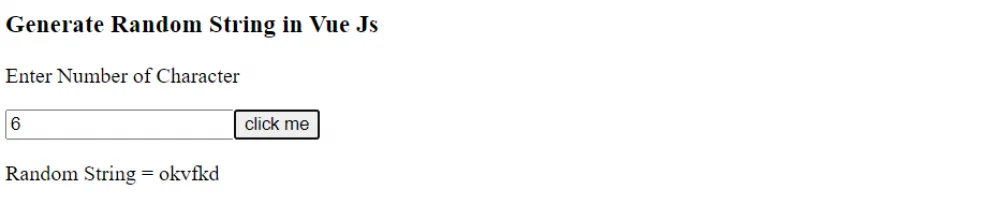
How to generate a random alphanumeric string in Vue.js?
In Vue.js, you can generate a random alphanumeric string by writing a function that uses Math.random() and the JavaScript string functions Math.floor(), string.length, and charAt().
Vue Js Generate Alpha Numeric String | Example
<div id="app">
<input type="number" v-model="strLength">
<button @click="myFunction">click me</button>
<p>Random Alphanumeric String = {{randomAlphanumeric}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
alphanumeric:'abcdefghijklmnopqrstuvwxyz0123456789',
strLength:"",
randomAlphanumeric:'',
}
},
methods:{
myFunction(){
this.randomAlphanumeric = ' ';
for(var i = 0; i <this.strLength;i++){
this.randomAlphanumeric += this.alphanumeric.charAt(Math.floor(Math.random()*this.alphanumeric.length))
}
},
}
}).mount('#app')
</script>
Output of above example
